Import “constants” could not be resolved(Python)
If you’re facing the error message “Import ‘constants’ could not be resolved” in your Python project, it indicates that Python is unable to locate the constants
module. Here’s how to troubleshoot and resolve this issue.
Step 1: Verify the Module Name
First, confirm that the module you’re trying to import is indeed named constants
. If it’s a local module, check that you have a file named constants.py
in your project directory.
Step 2: Check Your Directory Structure
Ensure your project structure is correct. For example:
/your_project_directory/
- env/ # Your virtual environment
- constants.py # Your constants file
- main.py # Your main script where you’re importing
Step 3: Use the Correct Import Statement
If constants.py
is in the same directory as your script, your import statement should simply be:
import constants
If constants.py
is in a folder, use:
from folder_name import constants
Step 4: Check the Python Path
To ensure that the current directory is included in your Python path, you can use the following code:
import sys print(sys.path)
If the current directory (.
) is not listed, you can add it:
if ‘.’ not in sys.path: sys.path.append(‘.’)
Step 5: Reinstall the Module
If constants
is an external module, reinstall it using these commands in your activated virtual environment:
pip uninstall constants pip install constants
Step 6: Restart Your IDE
Sometimes, the Integrated Development Environment (IDE) may not recognize changes immediately. Restart your IDE (like Visual Studio Code) after activating the virtual environment.
Step 7: Select the Correct Interpreter
Ensure your IDE is set to use the Python interpreter from your virtual environment. In Visual Studio Code, you can do this by:
- Clicking on the Python version in the bottom left corner.
- Selecting the interpreter associated with your virtual environment.
Step 8: Check for Typos
Python is case-sensitive, so check your import statement for any typos or incorrect casing.
Step 9: Look for Conflicting Files
Ensure there are no other files named constants.py
in your project directory that could be causing conflicts.
Final Solution Which Worked For Me!
Step1 : Open your VScode
Step2: Go to View Section

Step3 : Go to Command Pallete

Step 4 : Select Python Interpreter

Step5: In case, If you are using Virtual Environment,
click on Enter Interpreter Path

Step6 : Find

Step7: Browse .env File and click on select interpreter
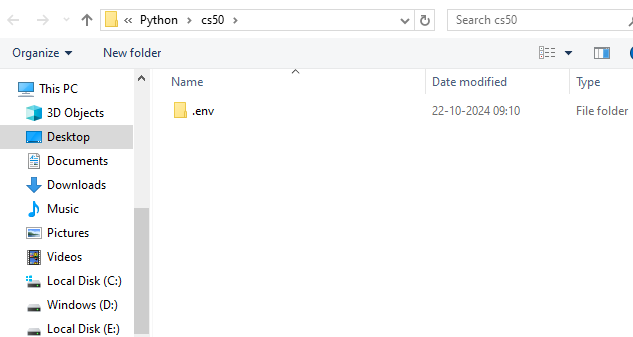
Step 8: Your Problem is Solved.
Conclusion
By following these steps, you should be able to resolve the “Import ‘constants’ could not be resolved” error. If the problem persists, consider sharing your directory structure, the code in your constants.py
file, and the exact import statement you’re using for further assistance.